Objective
After practicing with what we will explain to you, you should be able to create a Sprite using the tool that suits you best and incorporate it into FUSION-C to give it color and move it on the screen using the cursors.
In this example, we will work with the image editing software Gimp, as well as the tools we have already presented.
First of all, what is a Sprite?
A sprite is, in video games, a graphic element that can move on the screen. Initially, a sprite is partially transparent and can be animated (that is, it is composed of multiple overlapping bitmaps).
Designing a Sprite
For this guide, we have decided to create a sprite of a cat with dimensions of 16 x 16 pixels.
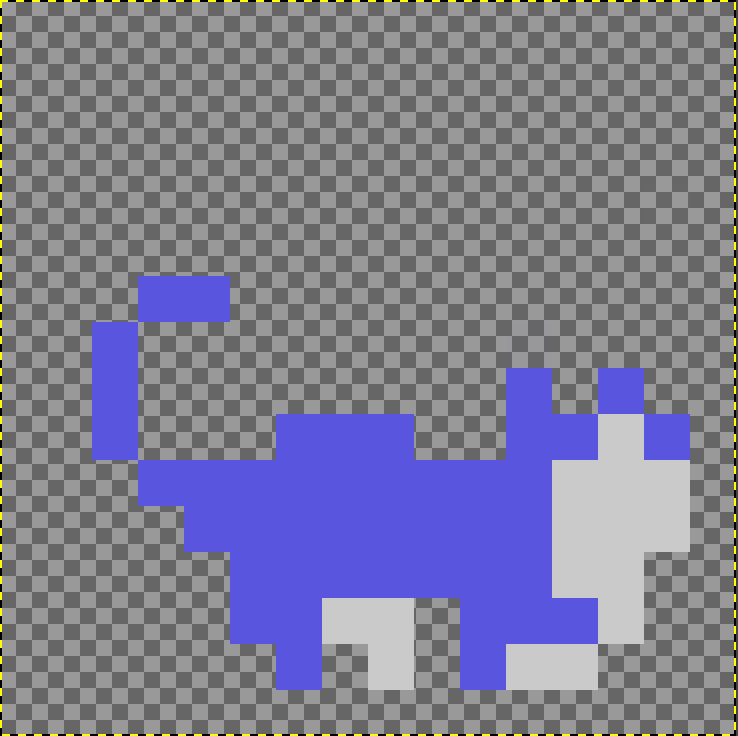
This is the result of drawing the cat using the Gimp program. To ensure that the cat sprite has a minimum amount of color detail, we have decided to create a sprite with two layers. One layer will contain the main color of the cat, while the other layer will depict the cat's spots. We will transfer this to the MSX as two sprites that will be moved simultaneously, creating the illusion of a single sprite.
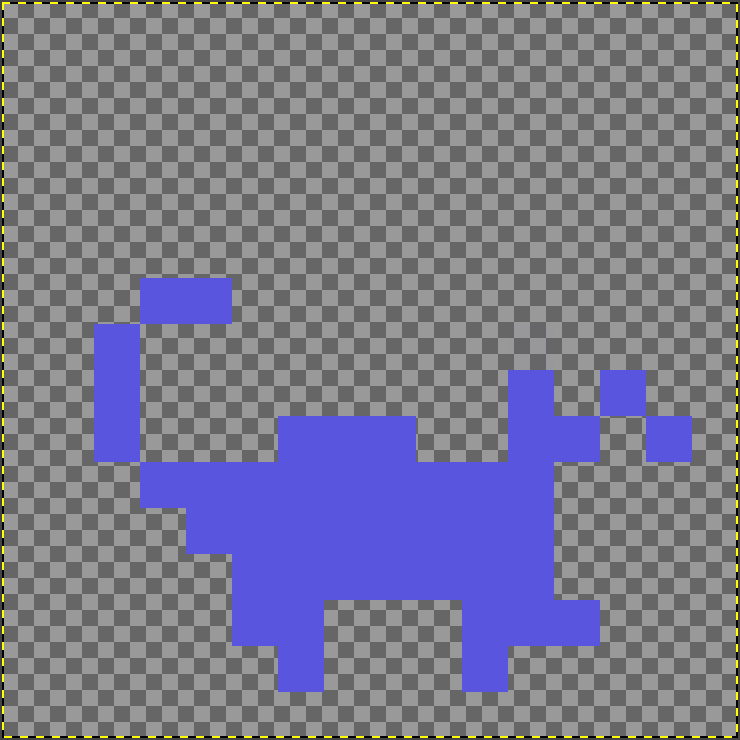
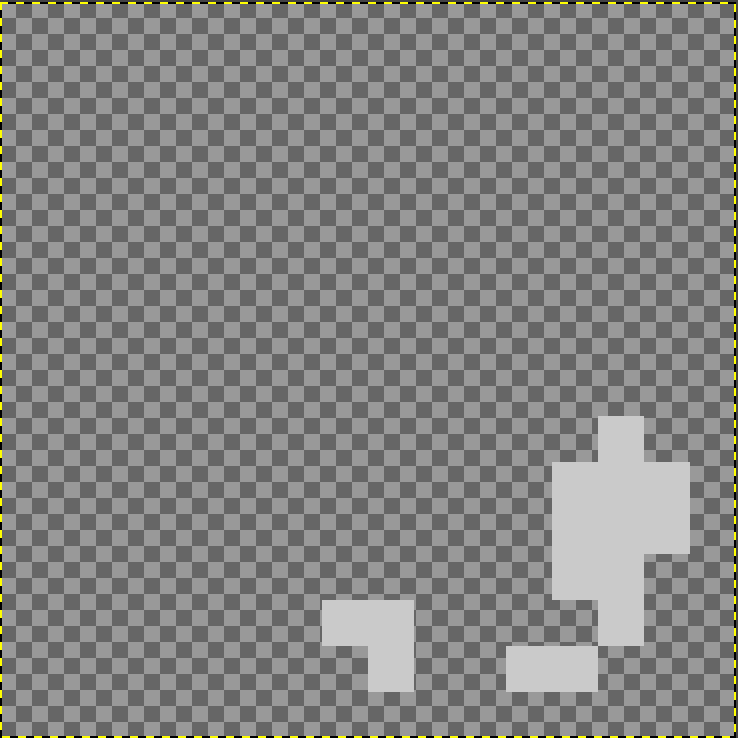
Next, I would like to mention a useful tool in Gimp that can help you draw sprites and then transfer the information into bits to be managed by the MSX.
This tool is a one-pixel grid that allows you to create your sprite designs comfortably within Gimp.
First, from the menu option "Image > Configure Grid," select a grid size of 1x1.
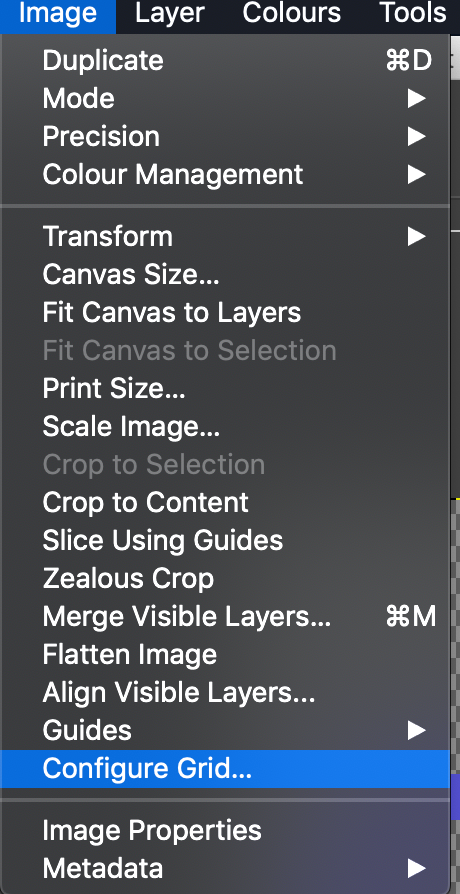
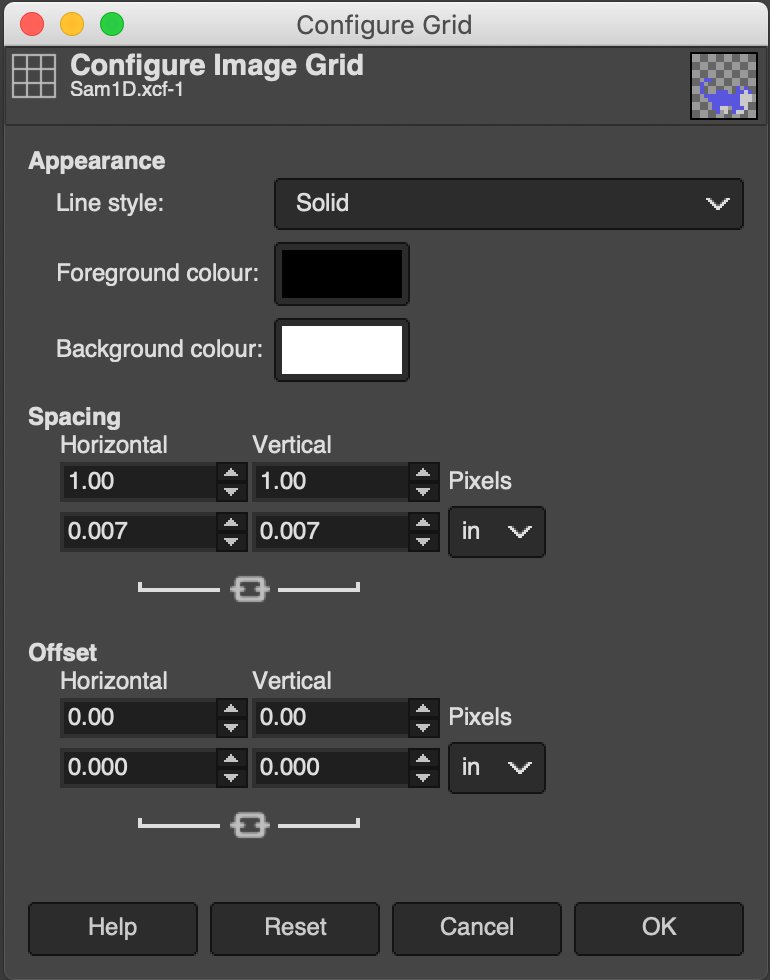
The grid will not appear until you activate it from the "View > Show Grid" menu option.
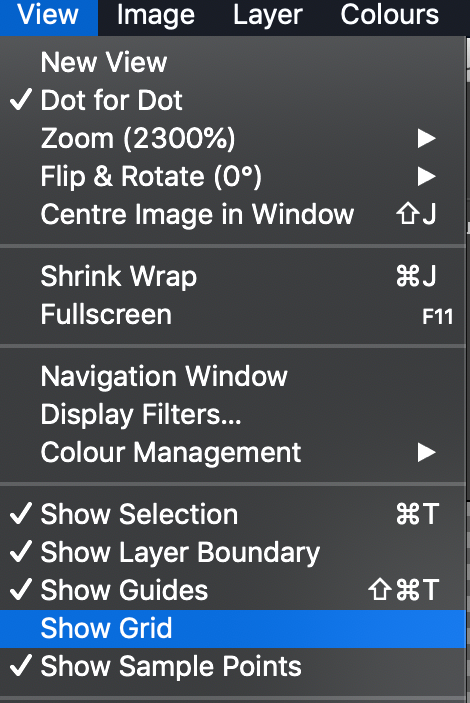
Once the grid is activated, it will be displayed on the image like this:
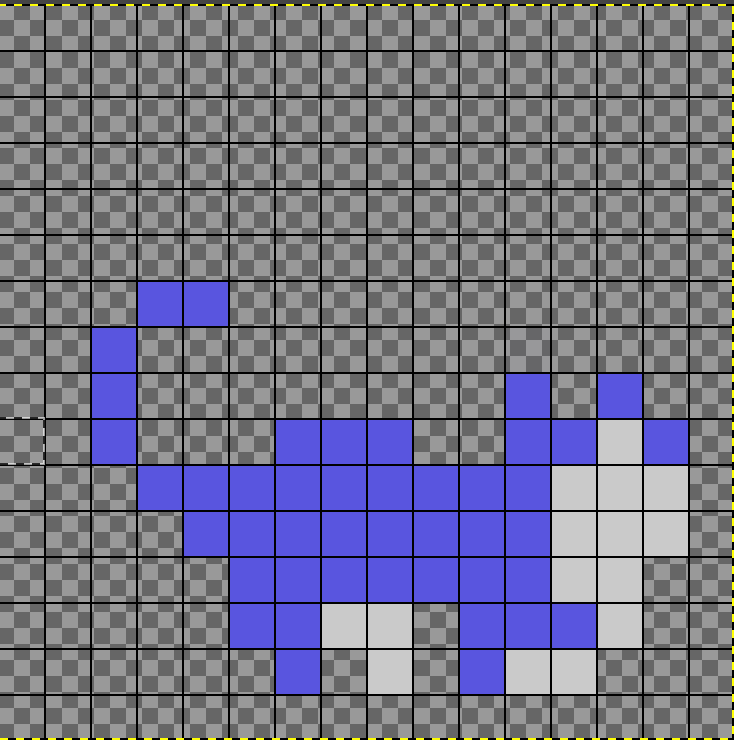
Each square of the grid corresponds to one pixel.
To create the cat, we have created a transparent image with dimensions of 16x16 pixels. By using the grid, we can design sprites with greater precision than with other tools.
If desired, we can also incorporate the 16-color palette of the MSX1 into Gimp. This palette can be found, for example, in Gimp format, at the following link [1].
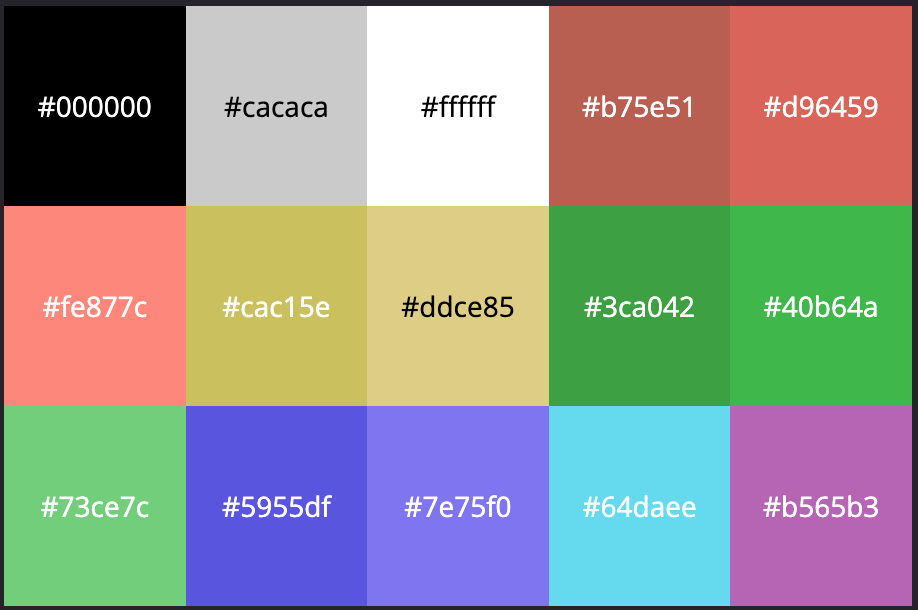
Designing the Animation
Now that we have introduced the design of the first sprite, let's design another sprite to animate the cat.
The concept behind animating a sprite is similar to traditional animation on paper, where multiple static positions are drawn and the sheets are rapidly flipped to create the illusion of animation.

In the image above, we can see a six frames animation of a cat. However, we will simplify it to two frames. When using sprites, a significant part of the management is handled by the VDP (Video Display Processor) as we are utilizing what is known as hardware sprites.
To generate the animation, we will switch between the two sprites on the screen while simultaneously moving the X coordinate.
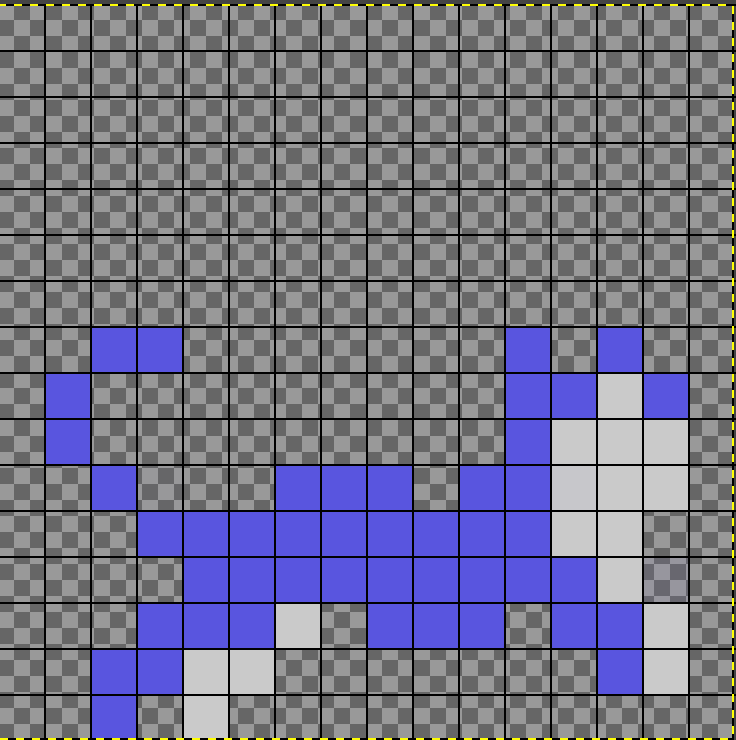
The image above is the second sprite of the animation. Similar to the first case, it consists of two sprites or a composite sprite with two layers.
Now that we have both cat sprites, as shown below, we can proceed to transfer this information to be used within FUSION-C for animation purposes. The animation will be achieved by overlaying the sprites and simultaneously moving them along the X coordinate.
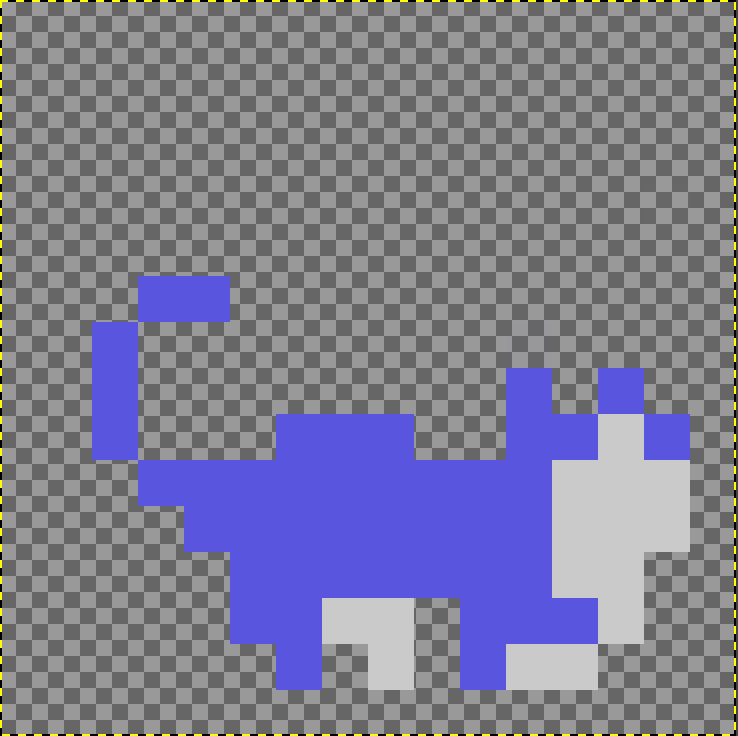
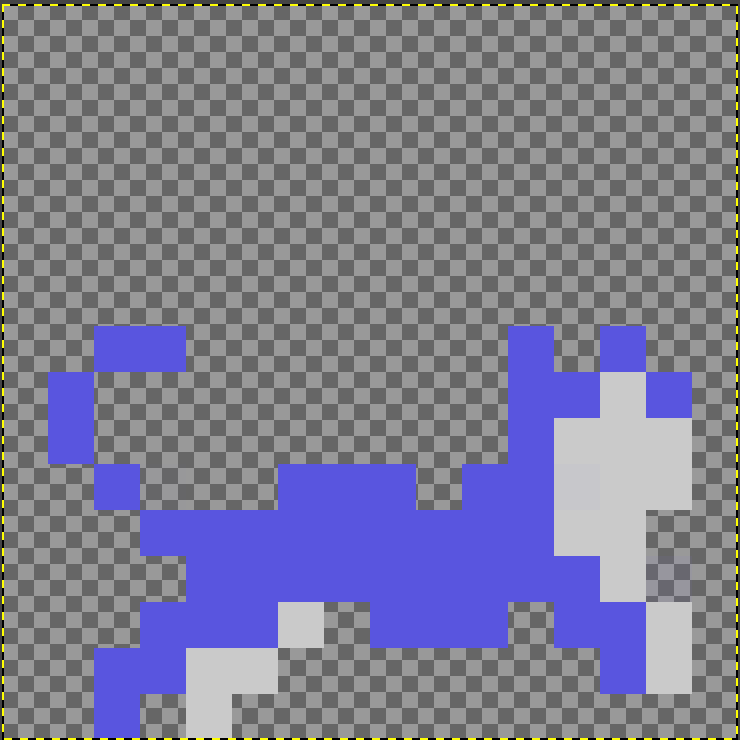
Converting what we have drawn into a language that the computer understands
Our sprites are 16 pixels wide and 16 pixels tall, meaning they have a size of 16x16. In FUSION-C, we have decided to transfer the information using 8x8 text patterns, dividing the sprite into four patterns as follows:
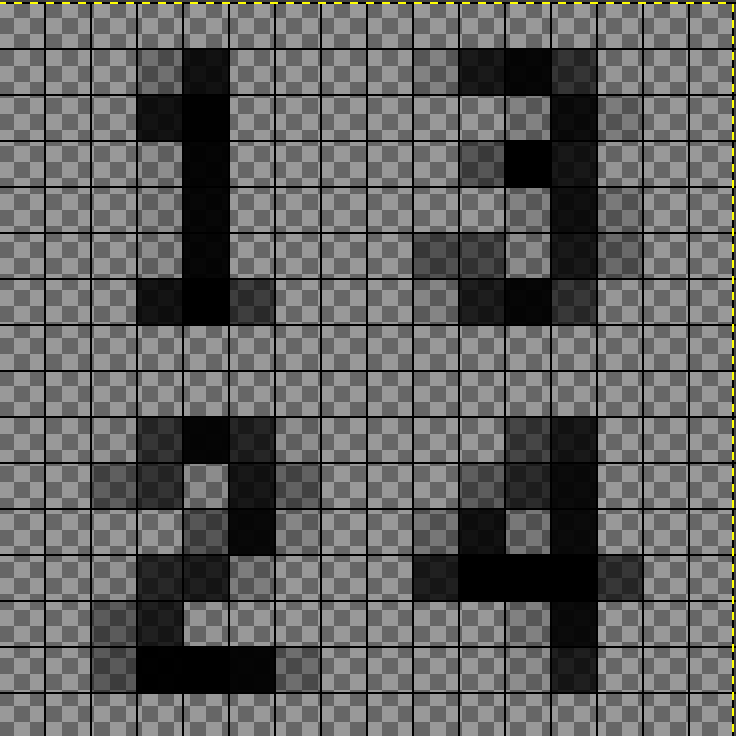
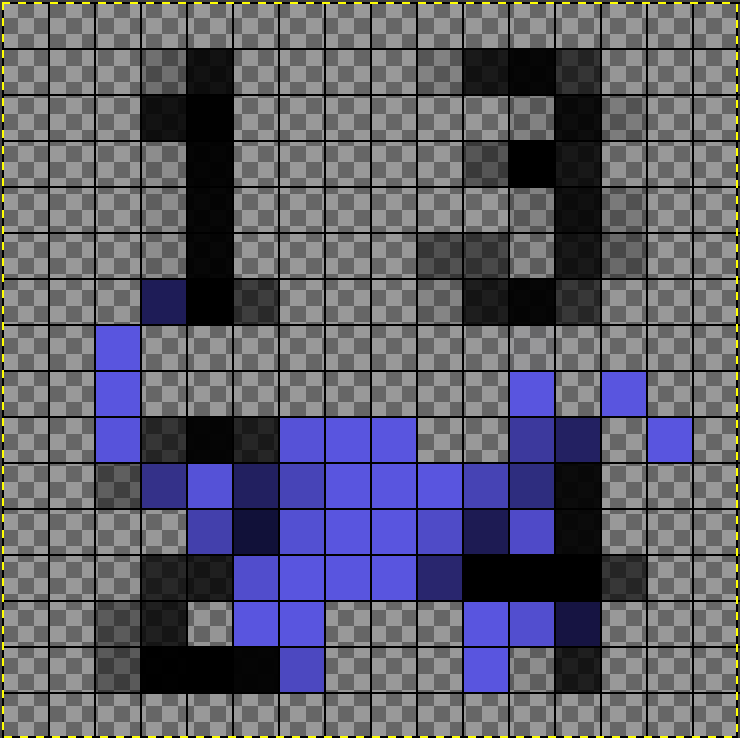
We will transfer the drawing information to the computer using the binary system. A 1 indicates the presence of a pixel, while a 0 indicates the absence of a pixel.
In the case of the first frame of the cat's movement, as we mentioned it consists of two layers or sprites, we will need 8 patterns of 8x8 to generate this frame.
The result transferred to FUSION-C of the first layer of the sprite for the first frame would be the four patterns shown below:
static const unsigned char body_idle_pattern_right_1[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00011000,
0b00100000
};
static const unsigned char body_idle_pattern_right_2[] = {
0b00100000,
0b00100011,
0b00011111,
0b00001111,
0b00000111,
0b00000110,
0b00000010,
0b00000000
};
static const unsigned char body_idle_pattern_right_3[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000
};
static const unsigned char body_idle_pattern_right_4[] = {
0b00010100,
0b10011010,
0b11110000,
0b11110000,
0b11110000,
0b00111000,
0b00100000,
0b00000000
};
In order to define the Sprite in FUSION-C, we need to use the function SetSpritePattern, to which we will pass three parameters: the pattern number, the pattern array, and the number of pattern lines.
For our first Sprite, which consists of the first layer of the first frame of the animation, it would be defined as shown below.
SetSpritePattern( 0, body_idle_pattern_right_1,8 );
SetSpritePattern( 1, body_idle_pattern_right_2,8 );
SetSpritePattern( 2, body_idle_pattern_right_3,8 );
SetSpritePattern( 3, body_idle_pattern_right_4,8 );
We still need to transfer the color information. Without the color information, our Sprite would appear as follows if we loaded it.
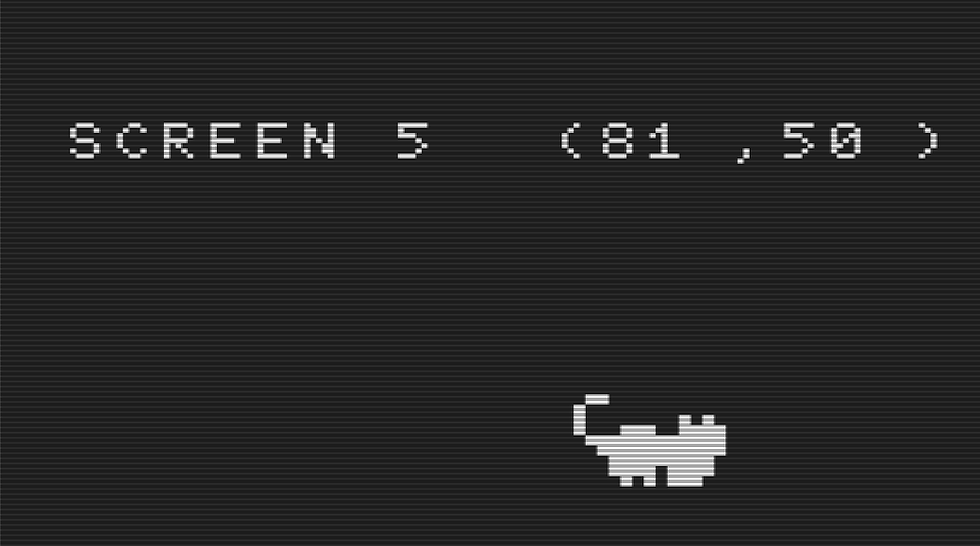
Defining the color palette that we will use.
First of all, we need to pass the color palette to be used in FUSION-C. In the case of MSX, it will be a palette of 16 colors. To do this, we will define an array where we will load 4-tuples of data in the following format:
MSX palette color number
Red component with decimals
Green component with decimals
Blue component with decimals
The values of R, G, and B can range from 0 to 7.
For our example, we have used the MSX1 color palette, which we will define in FUSION-C within an array as shown below.
char mypalette[] = {
0, 0,0,0, // transparent
1, 1,1,1, // black
2, 1.7,5,2, // medium green
3, 3.1,5.7,3.4, // light green
4, 2.4,2.3,6.1, // dark blue
5, 3.5,3.2,6.6, // light blue
6, 5,2.5,2.2, // dark red
7, 2.7,6,6.5, // cyan
8, 6,2.7,2.4, // medium red
9, 7,3.7,3.4, // light red
10, 5.6,5.3,2.5, // dark yellow
11, 6,5.7,3.7, // light yellow
12, 1.5,4.4,1.7, // dark green
13, 5,2.8,4.9, // magenta
14, 5.6,5.6,5.6, // gray
15, 7,7,7 // white
};
Coloring the Sprites
Once the color palette is defined, we need to transfer the color information to the sprites. To color the sprites, we will use the SC5SpriteColors function in FUSION-C. This function takes two parameters: the sprite number to be colored and an array that defines the color for each line of the sprite.
The arrays containing the color information for the sprite lines have the following format:
char LineColorsLayer4[16]= { 4,4,4,4,4,4,4,4,4,4,4,4,4,4,4,4 };
char LineColorsLayer14[16]= { 14,14,14,14,14,14,14,14,14,14,14,14,14,14,14,14 };
Here, we have defined the colors for the two layers or sprites of the first frame of the animation. The first layer takes the color "dark blue," and the second layer takes the color "gray" from the color palette defined above.
SC5SpriteColors(1,LineColorsLayer4);
SC5SpriteColors(2,LineColorsLayer14)
Here, we are loading the color information for the first and second sprites of the first frame of the animation, which correspond to the first and second layers of the sprite.
The game loop
In order for the program to constantly be aware of our actions, it needs to be running continuously. While it's running, in our particular case, the program will check if the ESC key has been pressed to exit, or if the arrow keys or the space bar have been pressed to move or make our sprite jump. As we progress, we'll revisit the concept of the game loop.
All the code that we're about to explain will be enclosed within this program loop, which runs indefinitely until the ESC key is pressed, which corresponds to the key code 27.
// Game loop
while (Inkey()!=27)
{
…
Screen(0);
Exit(0);
}
Moving the cat Sprite on the screen
We initialize the position of the cat sprite using the PutSprite function outside the game loop. The function takes the Sprite number, the starting pattern, the x and y position on the screen, and the sprite color as parameters. In MSX2, the color will be defined as we explained earlier, but this value won't have any effect.
x=79;
y=50;
PutSprite (1,0,x,y,4);
PutSprite (2,4,x,y,14);
The result of positioning the cat sprite at x=79 and y=50 would be as shown in the image below.
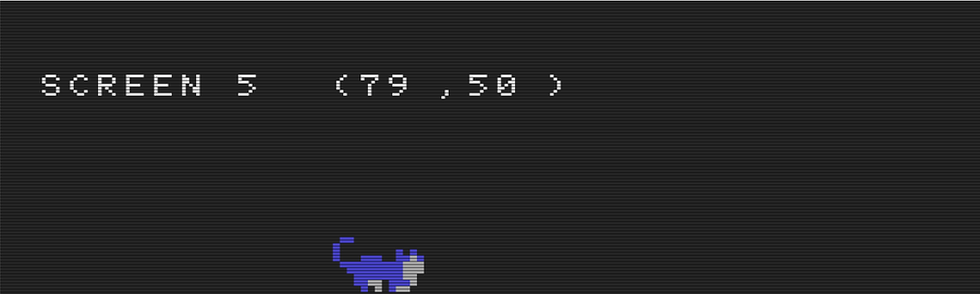
Now that we have the cat positioned, we need to make it move to the right or turn to the left when the left and right arrow keys are pressed. We achieve this with the code below.
// Game loop
while (Inkey()!=27)
{
stick = JoystickRead(0);
space = TriggerRead(0);
// Right
if(stick==3)
{
x=(x+1);
x=screen_limits_x(x);
if( x % 2 == 0)
{
PutSprite (1,0,x,y,4);
PutSprite (2,4,x,y,14);
}
if( x % 2 == 1)
{
PutSprite (1,8,x,y,4);
PutSprite (2,12,x,y,14);
}
FT_wait(10);
}
// Right
if(stick==7)
{
x=(x-1);
x=screen_limits_x(x);
if( x % 2 == 0)
{
PutSprite (1,16,x,y,4);
PutSprite (2,20,x,y,14);
}
if( x % 2 == 1)
{
PutSprite (1,24,x,y,4);
PutSprite (2,28,x,y,14);
}
FT_wait(10);
}
}
Screen(0);
Exit(0);
}
In the code snippet above, we can see the control of the cat's movement to the left and right.
In the function JoystickRead, if we pass the parameter 0, we will read the state of the pressed arrow keys. We will store the result of the function in the variable "stick" to determine the actions based on what we have pressed in the code below.
Analyzing the code snippet where stick=3, which indicates that the left arrow key is pressed, we can see how we change the set of two Sprites that make up a cat based on whether the x coordinate is odd or even. This is done using the integer division of the x coordinate by 2.
The possible values that "stick" can take are as follows.
0 | inactive |
1 | up |
2 | up + right |
3 | right |
4 | right + down |
5 | down |
6 | down + left |
7 | left |
8 | left + up |
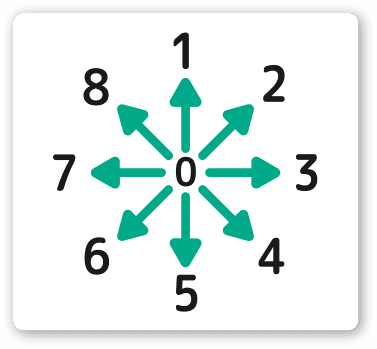
Next, I will provide you with the code listing as well as a link to a disk image so that you can run it directly.
//
// Fusion-C
// Example : Moving Sam Garcia The Cat 16x16 Sprites
//
#include "fusion-c/header/vdp_graph2.h"
#include "fusion-c/header/msx_fusion.h"
#include "fusion-c/header/vdp_sprites.h"
#include <stdio.h>
#include <string.h>
#include <math.h>
/* --------------------------------------------------------- */
/* SPRITEs of Sam Garcia The Cat */
/* ========================================================= */
static const unsigned char body_idle_pattern_right_1[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00011000,
0b00100000
};
static const unsigned char body_idle_pattern_right_2[] = {
0b00100000,
0b00100011,
0b00011111,
0b00001111,
0b00000111,
0b00000110,
0b00000010,
0b00000000
};
static const unsigned char body_idle_pattern_right_3[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000
};
static const unsigned char body_idle_pattern_right_4[] = {
0b00010100,
0b10011010,
0b11110000,
0b11110000,
0b11110000,
0b00111000,
0b00100000,
0b00000000
};
static const unsigned char detail_idle_pattern_right_1[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000
};
static const unsigned char detail_idle_pattern_right_2[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000001,
0b00000000,
0b00000000
};
static const unsigned char detail_idle_pattern_right_3[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000
};
static const unsigned char detail_idle_pattern_right_4[] = {
0b00000000,
0b00000100,
0b00001110,
0b00001110,
0b00001100,
0b10000100,
0b10011000,
0b00000000
};
static const unsigned char body_walk_pattern_right_1[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00110000
};
static const unsigned char body_walk_pattern_right_2[] = {
0b01000000,
0b01000001,
0b00100011,
0b00011111,
0b00001111,
0b00011100,
0b00110000,
0b00100000
};
static const unsigned char body_walk_pattern_right_3[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00010100
};
static const unsigned char body_walk_pattern_right_4[] = {
0b00011010,
0b00010000,
0b10110000,
0b11110000,
0b11111000,
0b11101100,
0b00000100,
0b00000000
};
static const unsigned char detail_walk_pattern_right_1[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000
};
static const unsigned char detail_walk_pattern_right_2[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000010,
0b00001100,
0b00001000
};
static const unsigned char detail_walk_pattern_right_3[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000
};
static const unsigned char detail_walk_pattern_right_4[] = {
0b00000100,
0b00001110,
0b00001110,
0b00001100,
0b00000100,
0b00000010,
0b00000010,
0b00000000
};
static const unsigned char body_idle_pattern_left_1[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000
};
static const unsigned char body_idle_pattern_left_2[] = {
0b00101000,
0b01011001,
0b00001111,
0b00001111,
0b00001111,
0b00011100,
0b00000100,
0b00000000
};
static const unsigned char body_idle_pattern_left_3[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00011000,
0b00000100
};
static const unsigned char body_idle_pattern_left_4[] = {
0b00000100,
0b11000100,
0b11111000,
0b11110000,
0b11100000,
0b01100000,
0b01000000,
0b00000000
};
static const unsigned char detail_idle_pattern_left_1[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000
};
static const unsigned char detail_idle_pattern_left_2[] = {
0b00000000,
0b00100000,
0b01110000,
0b01110000,
0b00110000,
0b00100001,
0b00011001,
0b00000000
};
static const unsigned char detail_idle_pattern_left_3[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000
};
static const unsigned char detail_idle_pattern_left_4[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b10000000,
0b00000000,
0b00000000
};
static const unsigned char body_walk_pattern_left_1[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00101000
};
static const unsigned char body_walk_pattern_left_2[] = {
0b01011000,
0b00001000,
0b00001101,
0b00001111,
0b00011111,
0b00110111,
0b00100000,
0b00000000
};
static const unsigned char body_walk_pattern_left_3[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00001100
};
static const unsigned char body_walk_pattern_left_4[] = {
0b00000010,
0b00000010,
0b11000100,
0b11111000,
0b11110000,
0b00111000,
0b00001100,
0b00000100
};
static const unsigned char detail_walk_pattern_left_1[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000
};
static const unsigned char detail_walk_pattern_left_2[] = {
0b00100000,
0b01110000,
0b01110000,
0b00110000,
0b00100000,
0b01000000,
0b01000000,
0b00000000
};
static const unsigned char detail_walk_pattern_left_3[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000
};
static const unsigned char detail_walk_pattern_left_4[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b01000000,
0b00110000,
0b00010000
};
static const unsigned char body_impuls_pattern_right_1[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00110000
};
static const unsigned char body_impuls_pattern_right_2[] = {
0b00100000,
0b00100000,
0b00100011,
0b00111111,
0b00011111,
0b00001111,
0b00001000,
0b00011000
};
static const unsigned char body_impuls_pattern_right_3[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00010100
};
static const unsigned char body_impuls_pattern_right_4[] = {
0b00011100,
0b00010100,
0b00100000,
0b11100000,
0b11100000,
0b11100000,
0b00100000,
0b00100000
};
static const unsigned char detail_impuls_pattern_right_1[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000
};
static const unsigned char detail_impuls_pattern_right_2[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000110,
0b00000100
};
static const unsigned char detail_impuls_pattern_right_3[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000
};
static const unsigned char detail_impuls_pattern_right_4[] = {
0b00000000,
0b00001000,
0b00011100,
0b00011100,
0b00011000,
0b00010000,
0b00011000,
0b00001000
};
static const unsigned char body_salt_pattern_right_1[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00100000
};
static const unsigned char body_salt_pattern_right_2[] = {
0b00100000,
0b00110000,
0b00110011,
0b00011111,
0b00001111,
0b00000110,
0b00001100,
0b00001000
};
static const unsigned char body_salt_pattern_right_3[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00101000,
0b00111000,
0b00110100
};
static const unsigned char body_salt_pattern_right_4[] = {
0b00100000,
0b01100000,
0b11100000,
0b11100000,
0b11100000,
0b00100000,
0b01100000,
0b00000000
};
static const unsigned char detail_salt_pattern_right_1[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000
};
static const unsigned char detail_salt_pattern_right_2[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000001,
0b00000010,
0b00000110
};
static const unsigned char detail_salt_pattern_right_3[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00001000
};
static const unsigned char detail_salt_pattern_right_4[] = {
0b00011100,
0b00011100,
0b00011100,
0b00011100,
0b00011000,
0b00001000,
0b00001000,
0b00000000
};
static const unsigned char body_impuls_pattern_left_1[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00101000
};
static const unsigned char body_impuls_pattern_left_2[] = {
0b00111000,
0b00101000,
0b00000100,
0b00000111,
0b00000111,
0b00000111,
0b00000100,
0b00000100
};
static const unsigned char body_impuls_pattern_left_3[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00001100
};
static const unsigned char body_impuls_pattern_left_4[] = {
0b00000100,
0b00000100,
0b10000100,
0b11111100,
0b11111000,
0b11110000,
0b00010000,
0b00011000
};
static const unsigned char detail_impuls_pattern_left_1[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000
};
static const unsigned char detail_impuls_pattern_left_2[] = {
0b00000000,
0b00010000,
0b00111000,
0b00111000,
0b00011000,
0b00001000,
0b00011000,
0b00010000
};
static const unsigned char detail_impuls_pattern_left_3[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000
};
static const unsigned char detail_impuls_pattern_left_4[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b01100000,
0b00100000
};
static const unsigned char body_salt_pattern_left_1[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00101000,
0b00111000,
0b00101100
};
static const unsigned char body_salt_pattern_left_2[] = {
0b00000100,
0b00000110,
0b00000111,
0b00000111,
0b00000111,
0b00000100,
0b00000110,
0b00000000
};
static const unsigned char body_salt_pattern_left_3[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000100
};
static const unsigned char body_salt_pattern_left_4[] = {
0b00000100,
0b00001100,
0b11001100,
0b11111000,
0b11110000,
0b01100000,
0b00110000,
0b00010000
};
static const unsigned char detail_salt_pattern_left_1[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00010000
};
static const unsigned char detail_salt_pattern_left_2[] = {
0b00111000,
0b00111000,
0b00111000,
0b00111000,
0b00011000,
0b00010000,
0b00010000,
0b00000000
};
static const unsigned char detail_salt_pattern_left_3[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000
};
static const unsigned char detail_salt_pattern_left_4[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b10000000,
0b01000000,
0b01100000
};
/* --------------------------------------------------------- */
/* SPRITE energia Sam Garcia The Cat */
/* ========================================================= */
static const unsigned char energia_pattern_1[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000
};
static const unsigned char energia_pattern_2[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00100000,
0b11000000,
0b11000000
};
static const unsigned char energia_pattern_3[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000
};
static const unsigned char energia_pattern_4[] = {
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000000,
0b00000100,
0b00000011,
0b00000011
};
// Functions prototypes
char FT_wait(int);
int screen_limits_x(int);
int screen_limits_y(int);
/* --------------------------------------------------------- */
char FT_wait(int cicles)
{
int i;
for(i=0;i<cicles;i++)
{
EnableInterupt();
Halt();
}
return(0);
}
int screen_limits_x(int x)
{
if( x < 0)
{
x=0;
}
if(x > 200)
{
x=200;
}
return x;
}
int screen_limits_y(int y)
{
if( y < 0)
{
y=0;
}
if(y > 160)
{
y=160;
}
return y;
}
/* --------------------------------------------------------- */
void main( void ) {
int x;
int y;
char strx[6];
char stry[6];
char stick;
char space;
char mypalette[] = {
0, 0,0,0, // transparent
1, 1,1,1, // black
2, 1.7,5,2, // medium green
3, 3.1,5.7,3.4, // light green
4, 2.4,2.3,6.1, // dark blue
5, 3.5,3.2,6.6, // light blue
6, 5,2.5,2.2, // dark red
7, 2.7,6,6.5, // cyan
8, 6,2.7,2.4, // medium red
9, 7,3.7,3.4, // light red
10, 5.6,5.3,2.5, // dark yellow
11, 6,5.7,3.7, // light yellow
12, 1.5,4.4,1.7, // dark green
13, 5,2.8,4.9, // magenta
14, 5.6,5.6,5.6, // gray
15, 7,7,7 // white
};
char LineColorsLayer4[16]= { 4,4,4,4,4,4,4,4,4,4,4,4,4,4,4,4 };
char LineColorsLayer14[16]= { 14,14,14,14,14,14,14,14,14,14,14,14,14,14,14,14 };
char LineColorsLayer12[16]= { 12,12,12,12,12,12,12,12,12,12,12,12,12,12,12,12 };
char LineColorsLayer3[16]= { 3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3 };
char LineColorsLayer2[16]= { 2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2 };
char LineColorsLayer1[16]= { 1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1 };
x=79;
y=50;
if(ReadMSXtype()==3) // IF MSX is Turbo-R Switch CPU to Z80 Mode
{
ChangeCPU(0);
}
SetColors(15,1,1);
Screen(5);
SetSC5Palette((Palette *)mypalette);
SpriteReset();
SpriteDouble();
// The 16 x 16 pixels Sprite is made of 4 patterns
SetSpritePattern( 0, body_idle_pattern_right_1,8 );
SetSpritePattern( 1, body_idle_pattern_right_2,8 );
SetSpritePattern( 2, body_idle_pattern_right_3,8 );
SetSpritePattern( 3, body_idle_pattern_right_4,8 );
SetSpritePattern( 4, detail_idle_pattern_right_1,8 );
SetSpritePattern( 5, detail_idle_pattern_right_2,8 );
SetSpritePattern( 6, detail_idle_pattern_right_3,8 );
SetSpritePattern( 7, detail_idle_pattern_right_4,8 );
SetSpritePattern( 8, body_walk_pattern_right_1,8 );
SetSpritePattern( 9, body_walk_pattern_right_2,8 );
SetSpritePattern( 10, body_walk_pattern_right_3,8 );
SetSpritePattern( 11, body_walk_pattern_right_4,8 );
SetSpritePattern( 12, detail_walk_pattern_right_1,8 );
SetSpritePattern( 13, detail_walk_pattern_right_2,8 );
SetSpritePattern( 14, detail_walk_pattern_right_3,8 );
SetSpritePattern( 15, detail_walk_pattern_right_4,8 );
SetSpritePattern( 16, body_idle_pattern_left_1,8 );
SetSpritePattern( 17, body_idle_pattern_left_2,8 );
SetSpritePattern( 18, body_idle_pattern_left_3,8 );
SetSpritePattern( 19, body_idle_pattern_left_4,8 );
SetSpritePattern( 20, detail_idle_pattern_left_1,8 );
SetSpritePattern( 21, detail_idle_pattern_left_2,8 );
SetSpritePattern( 22, detail_idle_pattern_left_3,8 );
SetSpritePattern( 23, detail_idle_pattern_left_4,8 );
SetSpritePattern( 24, body_walk_pattern_left_1,8 );
SetSpritePattern( 25, body_walk_pattern_left_2,8 );
SetSpritePattern( 26, body_walk_pattern_left_3,8 );
SetSpritePattern( 27, body_walk_pattern_left_4,8 );
SetSpritePattern( 28, detail_walk_pattern_left_1,8 );
SetSpritePattern( 29, detail_walk_pattern_left_2,8 );
SetSpritePattern( 30, detail_walk_pattern_left_3,8 );
SetSpritePattern( 31, detail_walk_pattern_left_4,8 );
SetSpritePattern( 32, body_impuls_pattern_right_1,8 );
SetSpritePattern( 33, body_impuls_pattern_right_2,8 );
SetSpritePattern( 34, body_impuls_pattern_right_3,8 );
SetSpritePattern( 35, body_impuls_pattern_right_4,8 );
SetSpritePattern( 36, detail_impuls_pattern_right_1,8 );
SetSpritePattern( 37, detail_impuls_pattern_right_2,8 );
SetSpritePattern( 38, detail_impuls_pattern_right_3,8 );
SetSpritePattern( 39, detail_impuls_pattern_right_4,8 );
SetSpritePattern( 40, energia_pattern_1,8 );
SetSpritePattern( 41, energia_pattern_2,8 );
SetSpritePattern( 42, energia_pattern_3,8 );
SetSpritePattern( 43, energia_pattern_4,8 );
SetSpritePattern( 44, body_salt_pattern_right_1,8 );
SetSpritePattern( 45, body_salt_pattern_right_2,8 );
SetSpritePattern( 46, body_salt_pattern_right_3,8 );
SetSpritePattern( 47, body_salt_pattern_right_4,8 );
SetSpritePattern( 48, detail_salt_pattern_right_1,8 );
SetSpritePattern( 49, detail_salt_pattern_right_2,8 );
SetSpritePattern( 50, detail_salt_pattern_right_3,8 );
SetSpritePattern( 51, detail_salt_pattern_right_4,8 );
SetSpritePattern( 52, body_impuls_pattern_left_1,8 );
SetSpritePattern( 53, body_impuls_pattern_left_2,8 );
SetSpritePattern( 54, body_impuls_pattern_left_3,8 );
SetSpritePattern( 55, body_impuls_pattern_left_4,8 );
SetSpritePattern( 56, detail_impuls_pattern_left_1,8 );
SetSpritePattern( 57, detail_impuls_pattern_left_2,8 );
SetSpritePattern( 58, detail_impuls_pattern_left_3,8 );
SetSpritePattern( 59, detail_impuls_pattern_left_4,8 );
SetSpritePattern( 60, body_salt_pattern_left_1,8 );
SetSpritePattern( 61, body_salt_pattern_left_2,8 );
SetSpritePattern( 62, body_salt_pattern_left_3,8 );
SetSpritePattern( 63, body_salt_pattern_left_4,8 );
SetSpritePattern( 64, detail_salt_pattern_left_1,8 );
SetSpritePattern( 65, detail_salt_pattern_left_2,8 );
SetSpritePattern( 66, detail_salt_pattern_left_3,8 );
SetSpritePattern( 67, detail_salt_pattern_left_4,8 );
SC5SpriteColors(1,LineColorsLayer4);
SC5SpriteColors(2,LineColorsLayer14);
SC5SpriteColors(3,LineColorsLayer12);
Sprite16();
// Printing initial coordinates on the Screen
PutText(0,10,"SCREEN 5",0);
sprintf(strx, "%i", x);
sprintf(stry, "%i", y);
PutText(83,10,"(",0);
PutText(91,10,strx,0);
PutText(113,10,",",0);
PutText(122,10,stry,0);
PutText(144,10,")",0);
// Positioning Sam on the Screen
PutSprite (1,0,x,y,4);
PutSprite (2,4,x,y,14);
// Game loop
while (Inkey()!=27)
{
stick = JoystickRead(0);
space = TriggerRead(0);
// Idle
if(stick!=0) {
sprintf(strx, "%i", x);
sprintf(stry, "%i", y);
PutText(83,10,"(",0);
PutText(91,10,strx,0);
PutText(113,10,",",0);
PutText(122,10,stry,0);
PutText(144,10,")",0);
}
// Up
if(stick==1)
{
y=(y-1);
PutSprite (1,0,x,y,4);
PutSprite (2,4,x,y,14);
FT_wait(10);
}
// Right
if(stick==3)
{
x=(x+1);
x=screen_limits_x(x);
if( x % 2 == 0)
{
PutSprite (1,0,x,y,4);
PutSprite (2,4,x,y,14);
}
if( x % 2 == 1)
{
PutSprite (1,8,x,y,4);
PutSprite (2,12,x,y,14);
}
FT_wait(10);
}
// Down
if(stick==5)
{
y=(y+1);
PutSprite (1,0,x,y,4);
PutSprite (2,4,x,y,14);
FT_wait(10);
}
// Right
if(stick==7)
{
x=(x-1);
x=screen_limits_x(x);
if( x % 2 == 0)
{
PutSprite (1,16,x,y,4);
PutSprite (2,20,x,y,14);
}
if( x % 2 == 1)
{
PutSprite (1,24,x,y,4);
PutSprite (2,28,x,y,14);
}
FT_wait(10);
}
// Button A
if(space==255 && stick==3)
{
PutSprite (1,32,x,y,4);
PutSprite (2,36,x,y,14);
FT_wait(10);
// Impulse
SC5SpriteColors(3,LineColorsLayer12);
PutSprite (3,40,x,y,12);
FT_wait(10);
SC5SpriteColors(3,LineColorsLayer2);
PutSprite (3,40,x,y,2);
FT_wait(10);
SC5SpriteColors(3,LineColorsLayer3);
PutSprite (3,40,x,y,3);
FT_wait(10);
SC5SpriteColors(3,LineColorsLayer1);
PutSprite (3,40,x,y,1);
FT_wait(10);
// Jump
x=x+20;
y=y-20;
PutSprite (1,44,x,y,4);
PutSprite (2,48,x,y,14);
FT_wait(10);
// Back to the floor
y=y+20;
PutSprite (1,0,x,y,4);
PutSprite (2,4,x,y,14);
}
// Button A
if(space==255 && stick==7)
{
PutSprite (1,52,x,y,4);
PutSprite (2,56,x,y,14);
FT_wait(10);
// Impulse
SC5SpriteColors(3,LineColorsLayer12);
PutSprite (3,40,x,y,12);
FT_wait(10);
SC5SpriteColors(3,LineColorsLayer2);
PutSprite (3,40,x,y,2);
FT_wait(10);
SC5SpriteColors(3,LineColorsLayer3);
PutSprite (3,40,x,y,3);
FT_wait(10);
SC5SpriteColors(3,LineColorsLayer1);
PutSprite (3,40,x,y,1);
FT_wait(10);
// Jump
x=x-20;
y=y-20;
PutSprite (1,60,x,y,4);
PutSprite (2,64,x,y,14);
FT_wait(10);
// Back to the floor
y=y+20;
PutSprite (1,16,x,y,4);
PutSprite (2,20,x,y,14);
}
}
Screen(0);
Exit(0);
}
Click here to see a working example.
Commentaires